Controls¶
Overview¶
Controls are the main building block for your forms for getting user data, diplaying information, and enforcing security. This section will cover the functions of each control in more detail, as well as the properties that can be used in your rules. For a brief overview of the form designer interface, see Form Designer.
The following are some common properties that can be modified with rules:
Note
In the subsections below, when a property is in this format
, that means it can be modified by a rule.
TextBox¶
This control accepts a small amount of text. This control is useful for when the user must submit raw text whether it is a single value, word, or phrase. Also allows for text to be masked for privacy. This field also allows for a default value by entering text into the field in the form designer. For large amounts of text, see Text Area.
A Text Box control has the following additional properties:
Placeholder: Text entered here will show as gray in the textbox in order to give the user a hint about what should enter.
Pattern: Uses JavaScript regular expressions to require a certain format for the user’s input.
Select Pattern: Some patterns have been provided for you. These patterns are email, SSN, and ZIP code
Max Characters: Takes a numerical value as the character limit for the user’s entry.
Masked: When checked, hides the user’s input. Useful for hiding paswords or other sensitive information.
value
: The text in the field. Accepts String values.
Text Area¶
This control records text from the user, similar to a text area; however this control supports multiple lines of texts, such as with paragraphs. This field also allows for a default value by entering text into the field in the form designer.
The Text Area control has the following additional properties:
Max Characters: Takes a numerical value as the character limit for the user’s entry.
Visible Lines: Takes a numerical value which determines how many lines can be visible at a time. This means larger values cause the Text Area control to take more vertical space.
value
: The text in the field. Takes String values.
Numeric¶
This control only allows numerical values, plus signs, and subtraction signs to be entered. This control also features an upward and downward arrow which allow the user to click to increment or decrement a value. Only integer values should be entered in these controls.
The numeric control has the following unique property:
value
: The numeric value of the field.
Currency¶
This control formats an input number as a monetary value, retaining two decimal points. Entering too many decimal points will reset the value to 0.00.
The currency control has the following unique property:
value
: represents the value of the field as a number.
Date¶
This control allows users to type in a date or select one from a calendar.
The date control has the following unique property:
value
: takes a date in the format of mm+'/'+dd+'/'+yyyy
Below is an example of getting the current date and assigning it to a date control:
var today = new Date();
var dd = today.getDate();
var mm = today.getMonth() + 1;
var yyyy = today.getFullYear();
if (dd<10) { dd='0'+dd; }
if (mm<10) { mm='0'+mm; }
Date100.value = mm+'/'+dd+'/'+yyyy;
Dropdown¶
This control gives the user a set of options to choose one from. Clicking the downward arrow displays the list of options. Typing a few letters brings the selection to the first word beginning with those letters.
The dropdown control has the following unique properties:
value
: holds the current dropdown’s selected value. Using a rule to assign a value that exists in the options list will set the dropdown to that value. Setting the value to be""
will clear the selection.options
: This is the list of the available value choices for the dropdown. In the form designer, each line represents an option, press enter to make a new line. For assigning options with rules, options are assigned with javascript dictionary objects.
An example of adding multiple options at once:
//The code below gives an options list with "cat" and "dog"
Dropdown.options = {'cat':'cat' , 'dog':'dog'};
An example of adding options one by one to a set. This can be useful if you are generating options from a database or using some other expression:
//initialize options variable
var options = {};
//populate option list with nine options
for(var i = 0; i < 9; i++){
//expression
options['Option ' + i] = 'Option' + i;
}
//put options into the control
Dropdown.options = options;
Checkbox¶
This control allows users to select multiple choices from a set of options.
Checkboxes have the following additional properties:
value
: Represents the currenly selected values. This takes the form of a JSON in string notation. Any values found in the JSON String will have the corressponding option checked; an empty JSON String or one that has values not in the options list will have nothing checked.Horizontal: Checking this option will align the items in rows instead of columns. When using a rule, this is called
inline
.options
: This is the list of the available value choices for the dropdown. In the form designer, each line represents an option, press enter to make a new line. For assigning options with rules, options are assigned with javascript dictionary objects, similar to dropdown controls.
An example of setting Checkbox options with a rule.
//CheckBox Options: Value 1=Option 1, Value 2=Option 2, Value 3=Option 3
//this line will leave only the Option 1 checked
Checkbox1.value = JSON.stringify(['Value 1']);
//This will check "Option 1" and "Option 3"
Checkbox1.value = JSON.stringify(['Value 1', 'Value 3']);
//This will leave nothing checked
Checkbox1.value = JSON.stringify([]);
Radio¶
Allows the user to pick a option from a set. Options are laid out in a list with a circle to the left. Selecting a circle selects the option and deselects the previously clicked option in the control. This is functionaly the same as a dropdown.
This control has the following additional properties:
value
: Holds the radio’s selected value. Using a rule to assign a value that exists in the options list will select that value. Setting the value to be""
will clear the selection.Horizontal: Checking this option will align the items in rows instead of columns.
inline
: This is how the horizontal property is accessed via form rules. It can either be true or false.options
: This is the list of the available value choices for the dropdown. In the form designer, each line represents an option, press enter to make a new line. For assigning options with rules, options are assigned with javascript dictionary objects.
Example rule to clear a selected option:
//removes radio selection
radio.value = "";
Note
The main difference between a radio and a dropdown is that users can not deselect a radio option, with the exception of something triggering the above rule.
Message¶
This control allows the form designer to add text or images to the form, whether it is a logo or some descriptive instructions. Messages are made with a rich text editor interface that allows you format your message with ease.
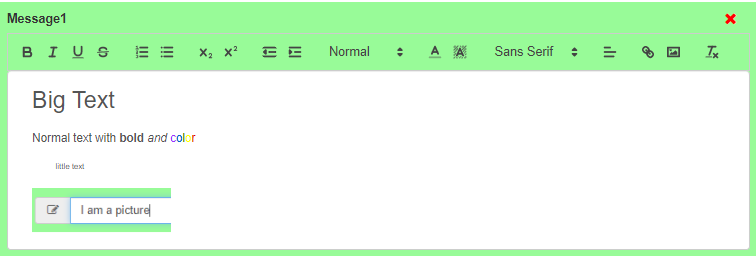
This control has the following additional properties:
value
: A property containing the message content.
Upload¶
This allows Users to upload documents as attachments to the form using their machine’s file explorer.
Link¶
Stores a link given in the form designer and opens a new tab using that link when clicked.
This control has the following properties:
URL
: Holds the link that the user will open.
Panel¶
This control acts as a container for other controls. Its security properties give the ability to selective show form content.
This control has the following properties:
Users: A username put here allows this specific user to view the control.
Roles: Any member of the role name put here will be able to view the panel and its contents.
Collapsible: Allows a user to shrink the panel by clicking the label bar.
collapsed
: If checked, the panel will be collapsed when opening the form.
Repeat¶
This control works very similar to a panel, however duplicates of the repeat, including all controls inside, can be made by clicking the green plus sign at the top right of the control. If there are multiple panels, clicking the minus sign remove a panel and its contents.
This control has the following properties:
Users: A username put here allows this specific user to view the control.
Roles: Any member of the role name put here will be able to view the Control and its contents.
Min: The user will not be able to delete panels so that there are less than this amount. The form will start with this many panels when opened.
Max: The user will not be able to add more than this many repeat panels.
Controls inside the repeat will also work slightly different with rules. Instead of a single
value
, Controls will instead use an array of values
, which starts counting from 0.
Below is an example of a rule with controls in repeats:
//This code gets the sum of values from numeric values in a repeat.
var sum = 0;
//for each numeric value from 0 to the end of the array
for(var i = 0; i < Numeric.values.length; i++){
//add value to sum
sum = sum + Numeric.values[i];
}
//store sum into a control on the form
Result.value = sum;
Signature¶
This allows users to draw a wet signature with a mouse or touchscreen.
The signature will be sent as an attached image file when the form is sent with an email or submitted.
Scan¶
This control is a clickable button which brings up the Scan Interface. From there you can scan in a new document using a connected scanner. Scanning additional documents Swill append them to documents in the preview. When using a connnected scanner, it will use the scanning interface from your scanner, so the options it presents may vary. A preview of the scanned document will show in the center of the scan interface. Once a document has been scanned, the user can save the document to their computer using the “Save” button. Clicking “Save Document” at the bottom right will attach it to the form. There is a load function as well where users can select a file from their local machine, however this will only take images from the selected documents. If you wish to get whole documents from a system, please use the upload control.
After saving the document to the form, the name of the document will appear under the scanner control to show what document was attached.
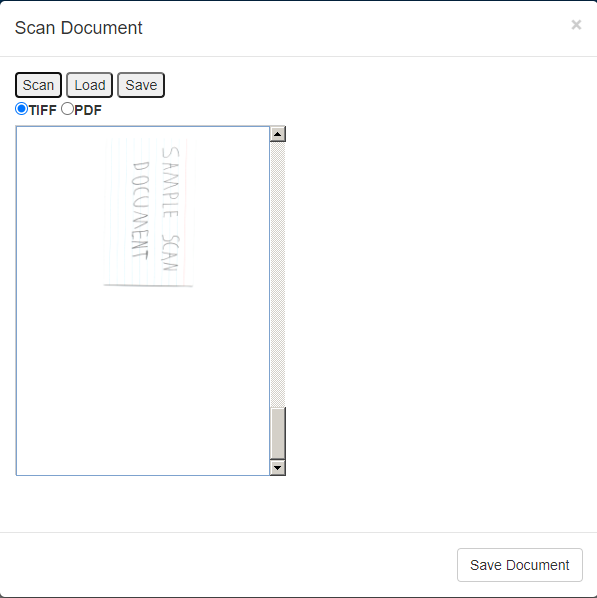
Trigger¶
This control is a clickable button which is used to activate rules. Clicking on this control is detectable with a change event or a click event. See Rules for more on making rules.
Payment¶
This control allows the user to perform a payment in the form. Upon clicking, users can be taken to a pop-out window to a site such as PayPal to handle payment processing. The Order Id for this payment will be automatically generated by LiveForms.
This control has the following additional properties:
Service: Used to specify which service will execute the payment process.
Merchant ID
: ID of the party recieving payment. This will be a business account registered with the payment service.Amount
: The dollar amount the user will be charged
There are currently three services available to connect with the payment control, PayPal, Authorize.net, and “Financial Payments” which connects to Herring Bank. It is advised that you test using the developer version of these services before giving acess to your users.
Note
PayPal offers a free testing environment which you can find at developer.paypal.com.
Captcha¶
The captcha control can be placed on a form in order to protect a form from automated form fillers. It simply requires a user to check the box before submitting the form. Users deemed as suspicious may have to perform aditional captcha tests.
This control has the following additional properties:
Center: Moves the captcha to the center of the control